A company website can be one of the greatest resources sending potential customers to you. To embed a form in a website is by far the most common thing you can find in a corporate website. Gone are the days of long queues to fill out a registration form or sign up for an event as we know online registration improves efficiencies and that saves us a lot of time. Email address is the most common mandatory field which is required in an online registration form. Are you struggling to collect valid email addresses for your websites?
In this tutorial, we demonstrate how to perform email validation for form submission in such a way that user will not submit the form without knowing of the errors in their input. To explain more, you can integrate the MailboxValidator email validation into your online form easily, to provide relevant feedback when your users enter an invalid email, blacklisted email, free email, and so on. This does not require a database.
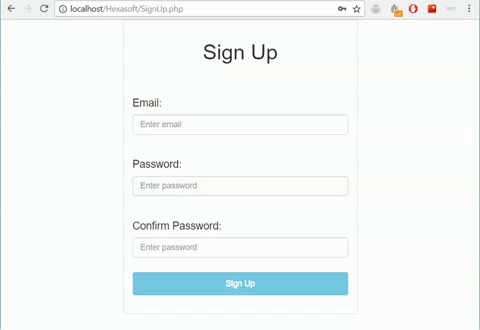
Step 1: Create simple online form (signup.php)
<html> <head> <title>Sign Up Page</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" type="text/css" href="css/bootstrap.min.css"> <link rel="stylesheet" href="css/bootstrap-theme.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="login_form.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/1000hz-bootstrap-validator/0.11.5/validator.min.js"></script> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" rel="stylesheet"></link> </head> <body> <div class="login"> <div class="login_container"> <div class="login_panel panel panel-default"> <div class="panel-body"> <h1 id="textHead" class="text-center">Sign Up</h1> <hr> <form data-toggle="validator" name="login_form" id="textContent" class="form-horizontal" method="post" action="su.php"> <div class="form-group"> <label class="control-label col-sm-3"></label> <div class="col-sm-12"> <h4>Email:</h4> <input type="text" data-error="Please enter your email field" class="form-control" name="email" placeholder="Enter email" id="email" required> <!--Check whether the field is empty--> <div class="help-block with-errors"></div> </div> </div> <p id="email"></p> <div class="form-group"> <label class="control-label col-sm-3"></label> <div class="col-sm-12"> <h4>Password:</h4> <!--Set the minimum length of password--> <input type="password" data-error="Please enter minimum of 6 characters." data-minlength="6" class="form-control" name="password" placeholder="Enter password" id="password" required> <!--Check whether the field is empty--> <div class="help-block with-errors"></div> </div> </div> <div class="form-group"> <label class="control-label col-sm-3"></label> <div class="col-sm-12"> <h4>Confirm Password:</h4> <input type="password" data-error="Please reenter password" class="form-control" name="password" placeholder="Enter password" id="password" data-match="#password" data-match-error="Password does not match" required> <!--Check whether the field is empty--> <div class="help-block with-errors"></div> </div> </div> <p id="password"></p> <div class="form-group"> <div class="col-sm-12"> <input type="Submit" class="btn btn-block btn-default btn-info" value="Sign Up"> </div> </div> </form> </div> </div> </div> </div> </body> </html>
Step 2: Create su.php file for calling MaiboxValidator API
<?php session_start(); $servername = "localhost"; $email = "root"; $password = ""; $database = ""; // Create connection to database $conn = new mysqli($servername, $email, $password,$database); $apiKey = 'Enter your API key'; $query = ''; if(!empty($_POST['email']) && !empty($_POST['password'])) { $email = $_POST['email']; $pass = $_POST['password']; $params['email']= $email; //Validate the email through MailboxValidator foreach($params as $key=>$value) { $query .= '&' . $key . '=' . rawurlencode($value); } $try = 0; do { //////////// //For https request, please make sure you have enabled php_openssl.dll extension. // //How to enable https //- Uncomment ;extension=php_openssl.dll by removing the semicolon in your php.ini, and restart the apache. // //In case you have difficulty to modify the php.ini, you can always make the http request instead of https. //////////// $result = file_get_contents('https://api.mailboxvalidator.com/v1/validation/single?key=' . $apiKey . $query); }while(!$result && $try++ < 3); $data = json_decode($result); //Check if query is success if ($data->is_syntax=='True') { //Get the data from database $query_email = "SELECT email FROM signup WHERE email='$email'"; $query_run_email = mysqli_query($conn,$query_email); //Check the input with database if (mysqli_num_rows($query_run_email)) { header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Email already exists")'; echo '</script>'; } else { //Insert data into database $sql = "INSERT INTO signup(email,password)VALUES ('$email', '$pass')"; mysqli_query($conn, $sql); //The message of account create successful header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Account created successful")'; echo '</script>'; } } else { //Error message of validation header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Account created failed, please enter a valid email!")'; echo '</script>'; } /* if need more information below is the example of error message else if ($data->is_free=='True') { header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Account created failed, free email are not supported!")'; echo '</script>'; } else if ($data->is_domain=='False') { header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Account created failed, please make sure your domain name is correct.")'; echo '</script>'; } else if ($data->is_suppressed == 'True') { header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Account created failed, your email address has been blacklisted!")'; echo '</script>'; } else if ($data->is_verified == 'False') { header( "refresh:0; url= signup.php" ); echo '<script language="javascript">'; echo 'alert("Account created failed, please enter a verified email!")'; echo '</script>'; } */ } ?>
Get started with MailboxValidator
Improve your email deliverability and sender reputation in email marketing.
Register today and clean your email lists for FREE!